Enhancing Your Business with a JavaScript Timestamp Converter
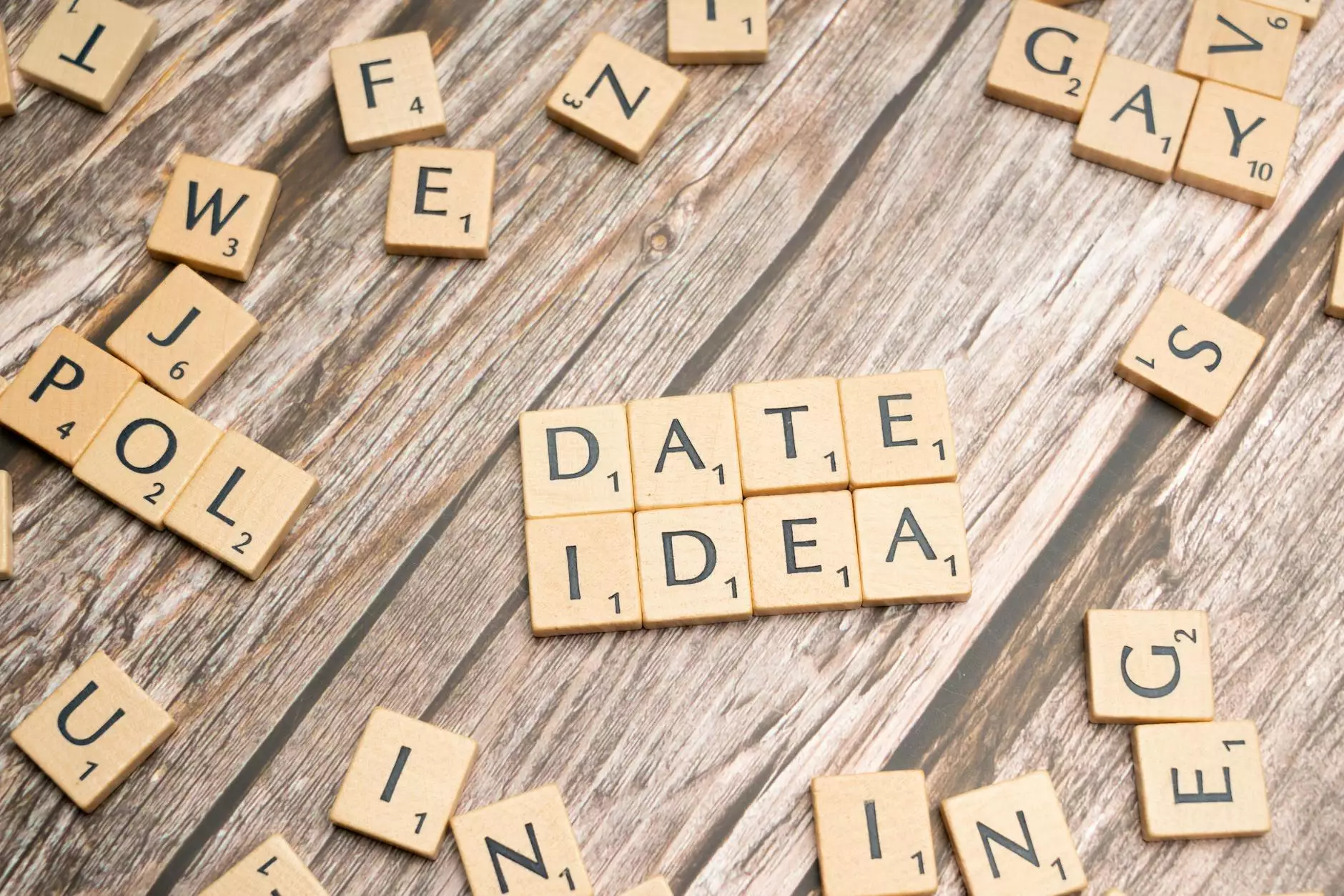
In the realm of web design and software development, understanding time handling is crucial. One common requirement is the conversion of timestamps. Whether dealing with timestamps from databases, APIs, or user input, having a reliable JavaScript timestamp converter can significantly improve your application's functionality and user experience. In this extensive guide, we will delve into the importance of timestamp conversion, its applications, and the technical aspects involved in implementing this feature effectively.
What is a Timestamp?
A timestamp is a sequence of characters or encoded information representing a specific time. Typically, it counts the number of seconds that have elapsed since a particular starting point, known as the “epoch.” For most systems and programming languages, this epoch is set to January 1, 1970. Timestamps are incredibly useful in various contexts, such as logging events, scheduling tasks, or tracking user interactions.
The Importance of JavaScript Timestamp Converter in Web Development
Every aspect of web development aims to enhance user experience and functionality. As developers, we often work with data that contains timestamps. A JavaScript timestamp converter helps interpret these dates and times in a human-readable format. Here are several reasons why a timestamp converter is essential:
- Improved User Experience: Presenting data in an easily understandable manner fosters more intuitive interactions.
- Data Consistency: Maintaining consistent timestamp formats across various systems and platforms is crucial for data integrity.
- Better Collaboration: Timestamps are often shared among team members or stakeholders; having a uniform format fosters better communication.
- Time Zone Management: Users from different geographic locations have different time zones. Handling these variations is made easier with a converter.
How Does a JavaScript Timestamp Converter Work?
A JavaScript timestamp converter processes the Unix timestamp, which is typically an integer representing the number of seconds since the epoch. Here's a breakdown of how this process works:
- Input Handling: The converter accepts a timestamp as input, which may be in seconds or milliseconds.
- Conversion Logic: Using JavaScript's built-in Date object, the converter calculates the corresponding date and time.
- Output Formatting: Finally, the output is formatted into a human-readable string, adjusting according to the user's timezone if necessary.
Implementing a JavaScript Timestamp Converter
Let’s create a simple yet efficient JavaScript timestamp converter that takes a timestamp and converts it to a readable date. Below is a step-by-step guide to coding this converter:
// Function to convert UNIX timestamp to human-readable date function convertTimestamp(timestamp) { // Check if the timestamp is in seconds and convert it to milliseconds if (timestamp.toString().length === 10) { timestamp *= 1000; } // Create a new Date object const date = new Date(timestamp); // Format the date into a human-readable format const options = { year: 'numeric', month: 'long', day: 'numeric', hour: '2-digit', minute: '2-digit', second: '2-digit', timeZoneName: 'short' }; return date.toLocaleString('en-US', options); } // Example usage const unixTimestamp = 1638316800; // Unix timestamp in seconds const readableDate = convertTimestamp(unixTimestamp); console.log("Readable Date:", readableDate);Benefits of Using a JavaScript Timestamp Converter
Integrating a JavaScript timestamp converter into your web applications provides numerous advantages:
- Efficiency: It automates the process of formatting dates, saving developers from writing repetitive functions.
- Accuracy: Automated conversion reduces the potential for human error when manually formatting dates.
- Customization: Developers can customize the output formats according to project requirements easily.
- Cross-Platform Compatibility: By using JavaScript, your converter will work seamlessly across different web browsers and platforms.
Common Use Cases for a JavaScript Timestamp Converter
There are various scenarios where a JavaScript timestamp converter proves vital:
1. Event Logging
In applications that track user activity or system events, timestamps enable precise logging of events. Implementing a converter allows administrators to review logs more effectively, interpreting timestamps in human-readable formats.
2. Scheduling Applications
For any functionality requiring scheduling, such as calendar applications or reminder settings, converting timestamps allows users to see their schedules clearly, avoiding any confusion over time calculations.
3. Data Analysis
Data analysts often work with datasets that include timestamps. A JavaScript converter helps transform raw numerical data into meaningful reports, assisting in identifying trends over time.
4. API Integration
When connecting to APIs that return Unix timestamps, utilizing a converter becomes essential for accurate data retrieval and display. This enhances communication between your application and third-party services.
Handling Time Zones with JavaScript Timestamp Converters
Time zone discrepancies can lead to significant confusion, especially for applications catering to a global audience. A well-designed JavaScript timestamp converter can handle time zone conversions effectively. Here’s how:
- Capture User Location: Use the Geolocation API to determine the user’s timezone based on their geographic location.
- Adjust Timestamps: Convert timestamps according to the retrieved timezone, ensuring the displayed dates reflect local times accurately.
Incorporating these techniques helps businesses provide a personalized user experience, leading to increased satisfaction and retention.
Conclusion: The Value of a JavaScript Timestamp Converter in Your Business
Ultimately, the importance of a JavaScript timestamp converter in modern web design and development cannot be overstated. It facilitates seamless interactions, enhances the accuracy of data representation, and improves overall user satisfaction. By implementing a robust converter, businesses like semalt.tools can leverage these benefits to strengthen their web applications, paving the way for operational efficiency and superior user experiences.
If you’re looking to optimize your applications and enhance functionalities, consider incorporating a reliable JavaScript timestamp converter. The investment in such a feature is guaranteed to yield dividends in both user engagement and application performance.
For more insights on web design and software development best practices, continue exploring the rich content available at semalt.tools.